Getting started
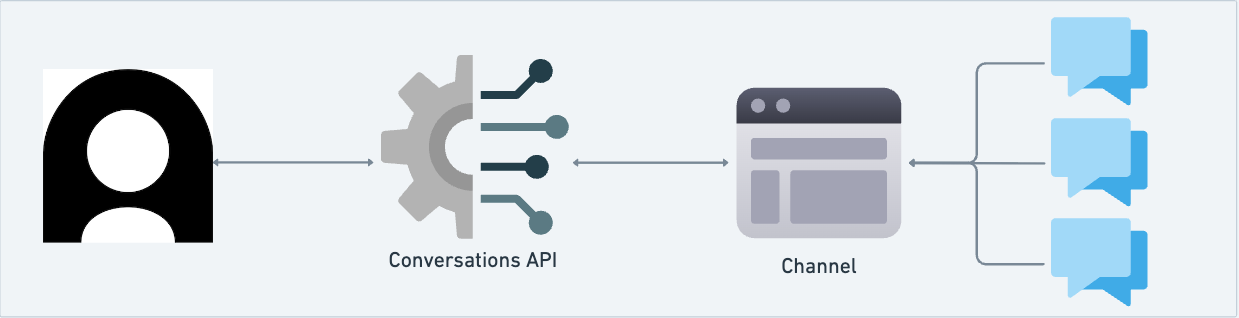
Who is this for?
Use this API to power your own messaging frontend, to integrate with your own email provider, or integrate your AI Agent in channels not otherwise supported out of the box by Ada.
Core concepts
Channel: A channel represents a communication pathway through which customers interact with your business for support. Custom channels created through the Conversations API are either messaging
or email
modality.
Conversation: A conversation is a sequence of messages exchanged between participants on a specific channel. Participants can be the end user, AI Agent, or human agents.
Message: Messages are authored by participants. The Conversations API supports text messages.
Webhook support
The Conversations API supports event notifications that enable you to track conversations and messages. The following events are currently supported:
v1.conversation.created
: triggered when a new conversation is created over a custom channel
v1.conversation.ended
: triggered when POST /v2/conversations/{conversation_id}/end/
is called from the Conversations API
v1.conversation.message
: triggered when a new message is added to a custom channel conversation
For more details on how to implement and use webhooks, refer to the webhook documentation.
Talk to your AI Agent over a custom channel
Setting up
Generate API key, if you don’t already have one
Create a webhook and subscribe to conversation events
1. Create a channel
Make sure you declare a modality
so that the AI Agent responds to users in the corresponding style.
Request example
2. Create a conversation over your channel
If you have an existing end user, include their End User ID in the payload.
Otherwise, the Conversations API will make a new end user automatically. Make sure you save the End User ID from the response so you can send messages from this End User in the next step.
When a conversation is successfully created, you’ll receive a v1.conversation.created
webhook event if subscribed.
Request example
3. Send a message on behalf of the End User
Request example
When a message is successfully sent, you’ll receive a v1.conversation.message
webhook event if subscribed. Note that the author.role
will be end_user
.
4. Listen for AI Agent response
When the AI Agent responds to your end user’s inquiry, you’ll receive a v1.conversation.message
webhook event if subscribed. Note that the author.role
will be ai_agent
.
5. End a conversation on behalf of an end user
To end a conversation on behalf of the end user, call